【Java】京淘项目Day04
京淘项目Day04
CV战士发布于 今天 06:06
京淘项目Day04
1.后端页面解析
1.1 页面布局说明
`<div id="cc" class="easyui-layout"><div data-options="region:'north',title:'North Title',split:true"></div>
<div data-options="region:'south',title:'South Title',split:true"></div>
<div data-options="region:'east',iconCls:'icon-reload',title:'East',split:true"></div>
<div data-options="region:'west',title:'West',split:true"></div>
<div data-options="region:'center',title:'center title'"></div>
</div>`
1.2 树形结构
`<div data-options="region:'west',title:'菜单',split:true"><ul class="easyui-tree">
<li>
<span>商品管理</span>
<ul>
<li>商品新增</li>
<li>商品修改</li>
<li>
<span>商品删除</span>
<ul>
<li>删除1</li>
<li>删除2</li>
</ul>
</li>
</ul>
</li>
<li>
<span>商品标题</span>
</li>
</ul>
</div>`
1.3 选项卡技术
`function addTab(title, url){if ($('#tt').tabs('exists', title)){
$('#tt').tabs('select', title);
} else {
var content = '<iframe scrolling="auto" frameborder="0" ></iframe>';
$('#tt').tabs('add',{
title:title,
content:content,
closable:true
});
}
}`
2.商品展现
2.1 JSON格式
2.1.1 什么是JSON
JSON(JavaScript Object Notation) 是一种轻量级的数据交换格式。它使得人们很容易的进行阅读和编写。同时也方便了机器进行解析和生成。它是基于 JavaScript Programming Language , Standard ECMA-262 3rd Edition - December 1999 的一个子集。 JSON采用完全独立于程序语言的文本格式,但是也使用了类C语言的习惯(包括C, C++, C#, Java, JavaScript, Perl, Python等)。这些特性使JSON成为理想的数据交换语言。
说明: JSON本质就是特殊格式的字符串.
2.1.2 对象格式
`{"key1":"value1","key2":"value2"}`
2.1.3 数组格式
`["value1","value2","value3"]`
2.1.4 嵌套格式
`[1,"tomcat猫",true,[1,2,[4,5,{"name":"京淘项目"}]],{"id":1,"name":"jerry","age":18}]`
2.2 商品数据信息
2.2.1 编辑POJO对象
2.2.2 实现商品列表说明
1). 页面标签
- 查询商品
2). 跳转页面信息
3).分析表格数据展现2.3 商品表格数据展现实现过程
### 2.3.1 页面结构说明
说明:该UI框架提供了一个规则,只要返回值是特定的格式要求,则会根据特定的属性展现具体的数据
`<div>
定义表格,并且通过url访问json数据, fitColumns:true表示自动适应,singleSelect:true 表示选中单个
<table class="easyui-datagrid" data-options="url:'datagrid_data.json',method:'get',fitColumns:true,singleSelect:false,pagination:true">
<thead>
<tr>
<th data-options="field:'code',width:100">Code</th>
<th data-options="field:'name',width:100">Name</th>
<th data-options="field:'price',width:100,align:'right'">Price</th>
</tr>
</thead>
</table>
</div>`
### 2.3.2 数据结构样式
### 2.3.3 编辑EasyUITable VO对象
`package com.jt.vo;
import com.jt.pojo.Item;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.experimental.Accessors;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
@Data
@Accessors(chain = true)
@NoArgsConstructor
@AllArgsConstructor
public class EasyUITable {
private Long total;
private List rows;
/**
* 对象转化为JSON时,必须对象的什么方法??? get方法
* JSON转化为对象时,调用的是对象的setxxx方法
*//*public String getJT(){
return "你好我是京淘";
}*/
}`
```
2.3 商品列表实现
----------
### 2.3.1 页面标识
说明:通过下列代码 可以获取URL地址: /item/query 返回值: 经过特殊格式封装的JSON数据
```
`<table class="easyui-datagrid" id="itemList" title="商品列表"
data-options="singleSelect:false,fitColumns:true,collapsible:true,pagination:true,url:'/item/query',method:'get',pageSize:20,toolbar:toolbar">
<thead>
<tr>
<th data-options="field:'ck',checkbox:true"></th>
<th data-options="field:'id',width:60">商品ID</th>
<th data-options="field:'title',width:200">商品标题</th>
<th data-options="field:'cid',width:100,align:'center',formatter:KindEditorUtil.findItemCatName">叶子类目</th>
<th data-options="field:'sellPoint',width:100">卖点</th>
<th data-options="field:'price',width:70,align:'right',formatter:KindEditorUtil.formatPrice">价格</th>
<th data-options="field:'num',width:70,align:'right'">库存数量</th>
<th data-options="field:'barcode',width:100">条形码</th>
<th data-options="field:'status',width:60,align:'center',formatter:KindEditorUtil.formatItemStatus">状态</th>
<th data-options="field:'created',width:130,align:'center',formatter:KindEditorUtil.formatDateTime">创建日期</th>
<th data-options="field:'updated',width:130,align:'center',formatter:KindEditorUtil.formatDateTime">更新日期</th>
</tr>
</thead>`
```
### 2.3.2 请求网址
说明:由于页面中添加了分页标签.所以在发起请求时,回默认的自动的拼接分页参数!!!
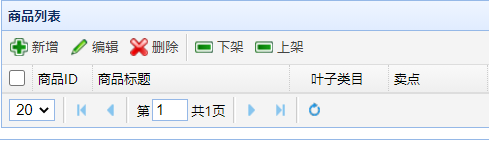
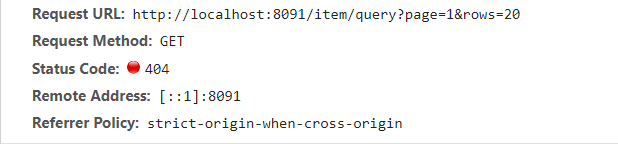
### 2.3.3 编辑ItemController
```
`package com.jt.controller;
import com.jt.vo.EasyUITable;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.jt.service.ItemService;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
import java.util.List;
@RestController //返回的数据都是JSON数据.
@RequestMapping("/item")
public class ItemController {
@Autowired
private ItemService itemService;
/**
* 业务说明: 完成商品分页查询
* URL地址: http://localhost:8091/item/query?page=1 页数 &rows=20 行数
* 参数: page/rows
* 返回值: EasyUITable
*/
@RequestMapping("/query")
public EasyUITable findItemByPage(Integer page,Integer rows){
return itemService.findItemByPage(page,rows);
}
}`
```
### 2.3.4 编辑ItemService
```
`package com.jt.service;
import com.jt.pojo.Item;
import com.jt.vo.EasyUITable;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.jt.mapper.ItemMapper;
import java.util.List;
@Service
public class ItemServiceImpl implements ItemService {
@Autowired
private ItemMapper itemMapper;
/**
* 1.方式1 查询所有的商品信息
* 2.方式2 自己能否手写sql实现分页查询
* 2.1 分页查询规则 sql: select * from tb_item limit 起始位置,记录数
* 第一页 SELECT * FROM tb_item LIMIT 0,20 [0,19] 共20条
* 第二页 SELECT * FROM tb_item LIMIT 20,20 [20,39]共20条
* 第三页 SELECT * FROM tb_item LIMIT 40,20 [40,59]共20条
* 第N页 SELECT * FROM tb_item LIMIT (page-1)*20,20 共20条
* @param page
* @param rows
* @return
*/
@Override
public EasyUITable findItemByPage(Integer page, Integer rows) {
//1.手写分页查询
//1.1计算起始位置
int start = (page-1)*rows;
List<Item> itemList = itemMapper.findItemByPage(start,rows);
//2.查询总记录数
long total = itemMapper.selectCount(null);
return new EasyUITable(total,itemList);
}
}`
```
### 2.3.5 编辑ItemMapper
```
`public interface ItemMapper extends BaseMapper<Item>{
/*Linux系统中严格区分大小写,所以表名及字段都必须与表一致...
* 老师为什么我的程序在windows中正常执行 ,linux中报错???
* */
@Select("SELECT * FROM tb_item ORDER BY updated DESC LIMIT #{start},#{rows}")
List<Item> findItemByPage(int start, Integer rows);
}`
```
### 2.3.6 页面效果展现
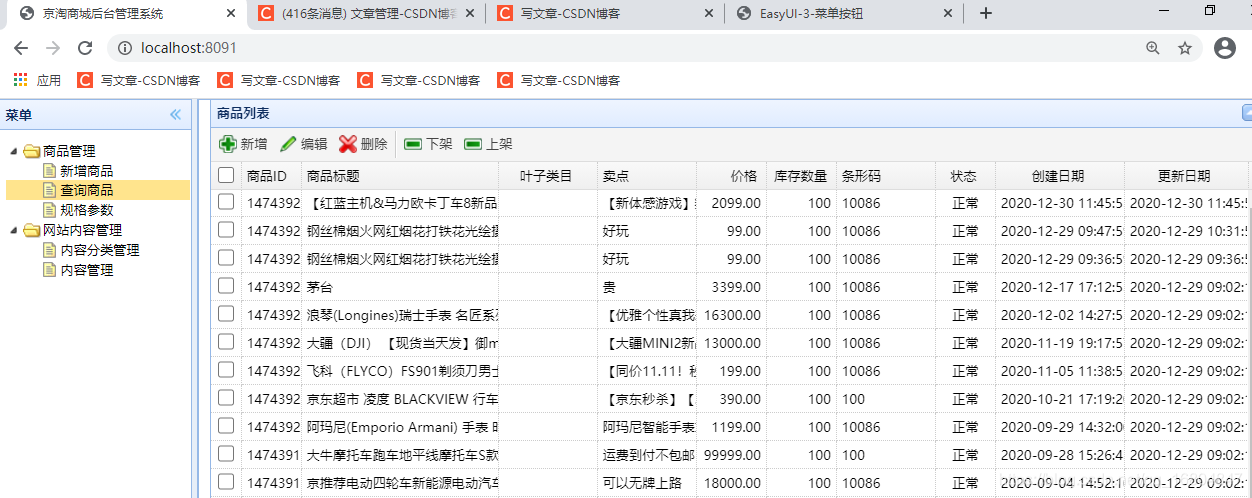
2.4 MP实现分页
----------
### 2.4.1 编辑ItemService
```
`/**
* MP分页相关说明:
* 1.构建一个分页对象(当前页,记录数,总记录数......)
* 2.为分页对象进行初始化操作
* 3.从分页操作中获取有效数据之后返回
* @param page
* @param rows
* @return
*/
@Override
public EasyUITable findItemByPage(Integer page, Integer rows) {
//1.定义分页对象 之后进行查询,
IPage<Item> iPage = new Page<>(page,rows);
//2.获取分页对象的其他数据
QueryWrapper<Item> queryWrapper = new QueryWrapper<>();
queryWrapper.orderByDesc("updated");
iPage = itemMapper.selectPage(iPage,queryWrapper);
long total = iPage.getTotal(); //获取总记录数
List<Item> itemList = iPage.getRecords(); //获取当前页的数据
return new EasyUITable(total,itemList);
}`
```
### 2.4.2 编辑分页配置类
```
`@Configuration //标识配置文件
public class MybatisPlusConfig {
@Bean //将数据交给spring容器管理
public PaginationInterceptor paginationInterceptor() {
PaginationInterceptor paginationInterceptor = new PaginationInterceptor();
// 设置请求的页面大于最大页后操作, true调回到首页,false 继续请求 默认false
paginationInterceptor.setOverflow(true);
// 设置最大单页限制数量,默认 500 条,-1 不受限制
// paginationInterceptor.setLimit(500);
// 开启 count 的 join 优化,只针对部分 left join
paginationInterceptor.setCountSqlParser(new JsqlParserCountOptimize(true));
return paginationInterceptor;
}
}`
```
2.5 数据格式化
---------
### 2.5.1 格式化价格
1).检查html代码
```
`<th data-options="field:'price',width:70,align:'right',formatter:KindEditorUtil.formatPrice">价格</th>`
```
2).检查函数
```
`/**
* val代表是当前节点的值
row代表当前的行级元素信息 对象
*/
// 格式化价格
formatPrice : function(val,row){
return (val/100).toFixed(2);
},`
```
### 2.5.2 格式化时间
1).编辑HTML代码
```
`<th data-options="field:'created',width:130,align:'center',formatter:KindEditorUtil.formatDateTime">创建日期</th>`
```
2).编辑页面JS
```
`// 格式化时间
formatDateTime : function(val,row){
//将字符串转化为js对象 时间对象
var now = new Date(val);
//将当前时间按照指定的格式进行转化
return now.format("yyyy-MM-dd hh:mm:ss");
},`
```
### 2.5.3 格式化商品状态
1).编辑HTML页面
```
`<th data-options="field:'status',width:60,align:'center',formatter:KindEditorUtil.formatItemStatus">状态</th>`
```
2).编辑页面JS
```
`// 格式化商品的状态
formatItemStatus : function formatStatus(val,row){
if (val == 1){
return '<span>上架</span>';
} else if(val == 2){
return '<span>下架</span>';
} else {
return '<span>未知</span>';
}
},`
```
### 2.5.4 JS引入过程
1).在父级页面中index.jsp中添加如下操作
```
`<jsp:include page="/commons/common-js.jsp"></jsp:include>`
```
2).检查common-js
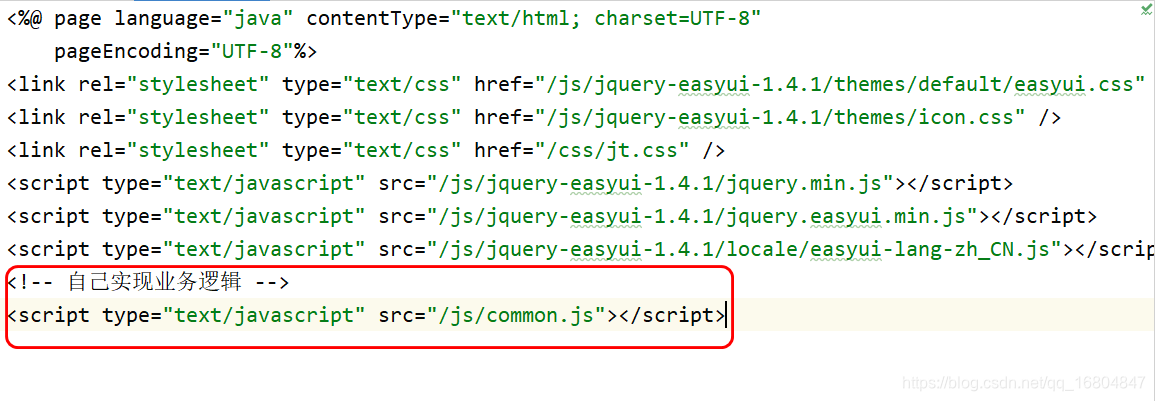
3.实现分类操作
========
3.1 实现商品分类回显
------------
### 3.1.1 编辑ItemCat对象
说明: 在jt-common中添加pojo对象
```
`package com.jt.pojo;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import lombok.Data;
import lombok.experimental.Accessors;
@TableName("tb_item_cat")
@Data
@Accessors(chain = true)
public class ItemCat extends BasePojo{
@TableId(type = IdType.AUTO)
private Long id; //商品分类id 主键自增
private Long parentId; //定义父级分类Id
private String name; //商品分类名称
private Integer status; //指定状态 1正常 2删除
private Integer sortOrder; //排序号
private Boolean isParent; //是否为父级
}`
```
### 3.1.1 页面标识
1).编辑html
```
`<th data-options="field:'cid',width:100,align:'center',formatter:KindEditorUtil.findItemCatName">叶子类目</th>`
```
2).编辑JS
```
`/**
* 完成$.ajax业务调用
属性说明:
1.type : 定义请求的类型 GET/POST/PUT/DELETE
2.URL: 指定请求的路径
3.dataType: 指定返回值类型 一般可以省略
4.data : ajax向后端服务器传递的数据
1.{key:value,key2:value2}
2.key=value&key2=value2
5.success: 设定回调函数一般都会携带参数
6.async 异步操作 默认值为true 改为false表示同步操作.
7.error 请求异常之后 执行的函数.
8.cache ajax是否使用缓存 默认值为true
1.动态获取用户传递的itemCatId的值.
2.利用ajax实现数据的动态的获取
ajax: $.get/$.post/$.getJSON/$.ajax
**/
findItemCatName : function(val,row){
//console.log("商品分类ID号:"+val);
let name = null;
// //异步出现了问题 由于异步操作程序没有返回数据之前,提前结束所以程序为null
$.ajax({
type : "GET",
url : "/itemCat/findItemCatById",
data : {id:val},
success : function(result){ //ItemCat
//console.log(result.name);
name = result.name;
},
async : false //设置为同步操作 true为异步....
})
return name;
},`
```
### 3.1.2 编辑ItemCatController
```
`package com.jt.controller;
import com.jt.pojo.ItemCat;
import com.jt.service.ItemCatService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/itemCat")
public class ItemCatController {
@Autowired
private ItemCatService itemCatService;
/**
* 实现商品分类查询
* url地址: http://localhost:8091/itemCat/findItemCatById?id=163
* 参数: id=163
* 返回值结果: itemCat对象
*/
@RequestMapping("/findItemCatById")
public ItemCat findItemCatById(Long id){
return itemCatService.findItemCatById(id);
}
}`
```
### 3.1.3 编辑ItemCatService
```
`package com.jt.service;
import com.jt.mapper.ItemCatMapper;
import com.jt.pojo.ItemCat;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class ItemCatServiceImpl implements ItemCatService{
@Autowired
private ItemCatMapper itemCatMapper;
@Override
public ItemCat findItemCatById(Long id) {
return itemCatMapper.selectById(id);
}
}`
```
### 3.1.4 页面效果展现
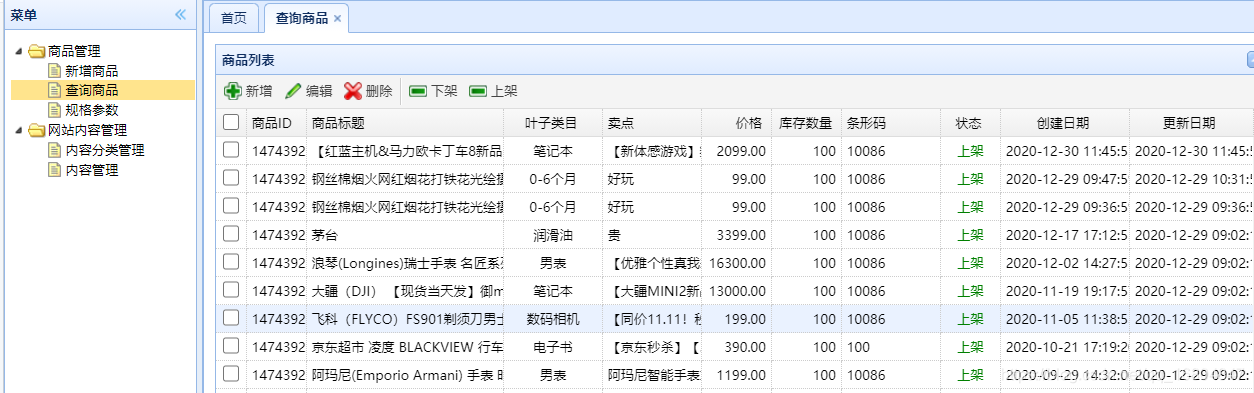
阅读 40更新于 今天 07:12
本作品系原创,采用《署名-非商业性使用-禁止演绎 4.0 国际》许可协议
CV战士
1 声望
6 粉丝
CV战士
1 声望
6 粉丝
宣传栏
目录
京淘项目Day04
1.后端页面解析
1.1 页面布局说明
`<div id="cc" class="easyui-layout"><div data-options="region:'north',title:'North Title',split:true"></div>
<div data-options="region:'south',title:'South Title',split:true"></div>
<div data-options="region:'east',iconCls:'icon-reload',title:'East',split:true"></div>
<div data-options="region:'west',title:'West',split:true"></div>
<div data-options="region:'center',title:'center title'"></div>
</div>`
1.2 树形结构
`<div data-options="region:'west',title:'菜单',split:true"><ul class="easyui-tree">
<li>
<span>商品管理</span>
<ul>
<li>商品新增</li>
<li>商品修改</li>
<li>
<span>商品删除</span>
<ul>
<li>删除1</li>
<li>删除2</li>
</ul>
</li>
</ul>
</li>
<li>
<span>商品标题</span>
</li>
</ul>
</div>`
1.3 选项卡技术
`function addTab(title, url){if ($('#tt').tabs('exists', title)){
$('#tt').tabs('select', title);
} else {
var content = '<iframe scrolling="auto" frameborder="0" ></iframe>';
$('#tt').tabs('add',{
title:title,
content:content,
closable:true
});
}
}`
2.商品展现
2.1 JSON格式
2.1.1 什么是JSON
JSON(JavaScript Object Notation) 是一种轻量级的数据交换格式。它使得人们很容易的进行阅读和编写。同时也方便了机器进行解析和生成。它是基于 JavaScript Programming Language , Standard ECMA-262 3rd Edition - December 1999 的一个子集。 JSON采用完全独立于程序语言的文本格式,但是也使用了类C语言的习惯(包括C, C++, C#, Java, JavaScript, Perl, Python等)。这些特性使JSON成为理想的数据交换语言。
说明: JSON本质就是特殊格式的字符串.
2.1.2 对象格式
`{"key1":"value1","key2":"value2"}`
2.1.3 数组格式
`["value1","value2","value3"]`
2.1.4 嵌套格式
`[1,"tomcat猫",true,[1,2,[4,5,{"name":"京淘项目"}]],{"id":1,"name":"jerry","age":18}]`
2.2 商品数据信息
2.2.1 编辑POJO对象
2.2.2 实现商品列表说明
1). 页面标签
- 查询商品
2). 跳转页面信息
3).分析表格数据展现2.3 商品表格数据展现实现过程
### 2.3.1 页面结构说明
说明:该UI框架提供了一个规则,只要返回值是特定的格式要求,则会根据特定的属性展现具体的数据
`<div>
定义表格,并且通过url访问json数据, fitColumns:true表示自动适应,singleSelect:true 表示选中单个
<table class="easyui-datagrid" data-options="url:'datagrid_data.json',method:'get',fitColumns:true,singleSelect:false,pagination:true">
<thead>
<tr>
<th data-options="field:'code',width:100">Code</th>
<th data-options="field:'name',width:100">Name</th>
<th data-options="field:'price',width:100,align:'right'">Price</th>
</tr>
</thead>
</table>
</div>`
### 2.3.2 数据结构样式
### 2.3.3 编辑EasyUITable VO对象
`package com.jt.vo;
import com.jt.pojo.Item;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.experimental.Accessors;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.List;
@Data
@Accessors(chain = true)
@NoArgsConstructor
@AllArgsConstructor
public class EasyUITable {
private Long total;
private List rows;
/**
* 对象转化为JSON时,必须对象的什么方法??? get方法
* JSON转化为对象时,调用的是对象的setxxx方法
*//*public String getJT(){
return "你好我是京淘";
}*/
}`
```
2.3 商品列表实现
----------
### 2.3.1 页面标识
说明:通过下列代码 可以获取URL地址: /item/query 返回值: 经过特殊格式封装的JSON数据
```
`<table class="easyui-datagrid" id="itemList" title="商品列表"
data-options="singleSelect:false,fitColumns:true,collapsible:true,pagination:true,url:'/item/query',method:'get',pageSize:20,toolbar:toolbar">
<thead>
<tr>
<th data-options="field:'ck',checkbox:true"></th>
<th data-options="field:'id',width:60">商品ID</th>
<th data-options="field:'title',width:200">商品标题</th>
<th data-options="field:'cid',width:100,align:'center',formatter:KindEditorUtil.findItemCatName">叶子类目</th>
<th data-options="field:'sellPoint',width:100">卖点</th>
<th data-options="field:'price',width:70,align:'right',formatter:KindEditorUtil.formatPrice">价格</th>
<th data-options="field:'num',width:70,align:'right'">库存数量</th>
<th data-options="field:'barcode',width:100">条形码</th>
<th data-options="field:'status',width:60,align:'center',formatter:KindEditorUtil.formatItemStatus">状态</th>
<th data-options="field:'created',width:130,align:'center',formatter:KindEditorUtil.formatDateTime">创建日期</th>
<th data-options="field:'updated',width:130,align:'center',formatter:KindEditorUtil.formatDateTime">更新日期</th>
</tr>
</thead>`
```
### 2.3.2 请求网址
说明:由于页面中添加了分页标签.所以在发起请求时,回默认的自动的拼接分页参数!!!
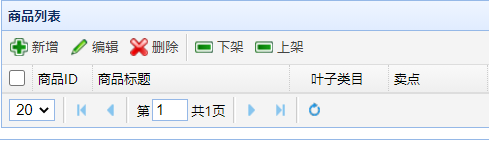
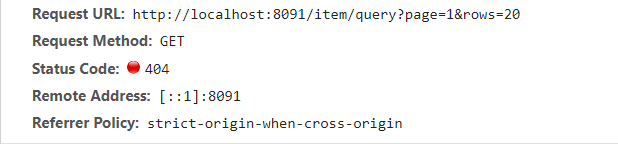
### 2.3.3 编辑ItemController
```
`package com.jt.controller;
import com.jt.vo.EasyUITable;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.jt.service.ItemService;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
import java.util.List;
@RestController //返回的数据都是JSON数据.
@RequestMapping("/item")
public class ItemController {
@Autowired
private ItemService itemService;
/**
* 业务说明: 完成商品分页查询
* URL地址: http://localhost:8091/item/query?page=1 页数 &rows=20 行数
* 参数: page/rows
* 返回值: EasyUITable
*/
@RequestMapping("/query")
public EasyUITable findItemByPage(Integer page,Integer rows){
return itemService.findItemByPage(page,rows);
}
}`
```
### 2.3.4 编辑ItemService
```
`package com.jt.service;
import com.jt.pojo.Item;
import com.jt.vo.EasyUITable;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.jt.mapper.ItemMapper;
import java.util.List;
@Service
public class ItemServiceImpl implements ItemService {
@Autowired
private ItemMapper itemMapper;
/**
* 1.方式1 查询所有的商品信息
* 2.方式2 自己能否手写sql实现分页查询
* 2.1 分页查询规则 sql: select * from tb_item limit 起始位置,记录数
* 第一页 SELECT * FROM tb_item LIMIT 0,20 [0,19] 共20条
* 第二页 SELECT * FROM tb_item LIMIT 20,20 [20,39]共20条
* 第三页 SELECT * FROM tb_item LIMIT 40,20 [40,59]共20条
* 第N页 SELECT * FROM tb_item LIMIT (page-1)*20,20 共20条
* @param page
* @param rows
* @return
*/
@Override
public EasyUITable findItemByPage(Integer page, Integer rows) {
//1.手写分页查询
//1.1计算起始位置
int start = (page-1)*rows;
List<Item> itemList = itemMapper.findItemByPage(start,rows);
//2.查询总记录数
long total = itemMapper.selectCount(null);
return new EasyUITable(total,itemList);
}
}`
```
### 2.3.5 编辑ItemMapper
```
`public interface ItemMapper extends BaseMapper<Item>{
/*Linux系统中严格区分大小写,所以表名及字段都必须与表一致...
* 老师为什么我的程序在windows中正常执行 ,linux中报错???
* */
@Select("SELECT * FROM tb_item ORDER BY updated DESC LIMIT #{start},#{rows}")
List<Item> findItemByPage(int start, Integer rows);
}`
```
### 2.3.6 页面效果展现
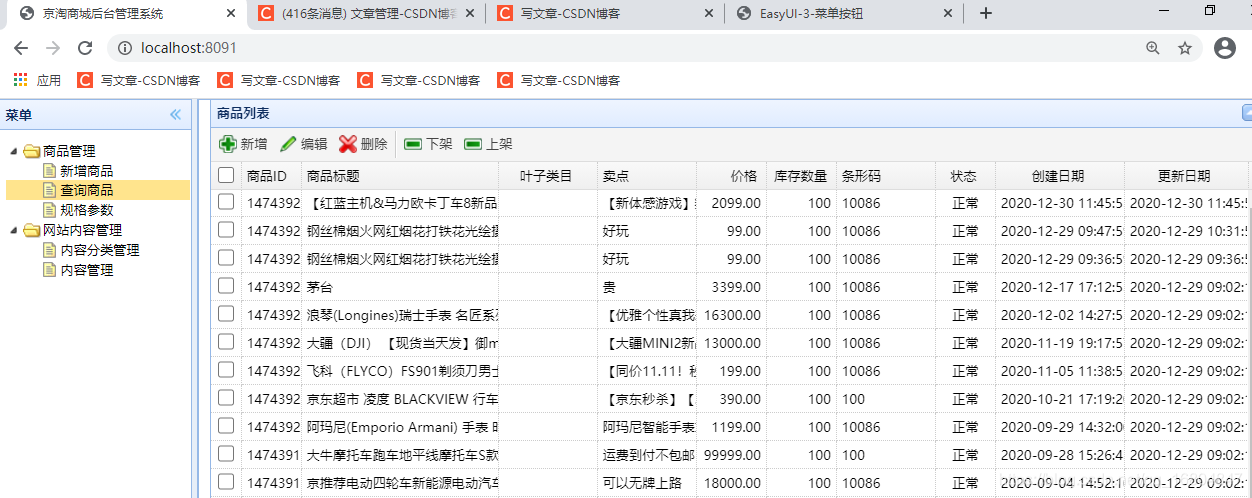
2.4 MP实现分页
----------
### 2.4.1 编辑ItemService
```
`/**
* MP分页相关说明:
* 1.构建一个分页对象(当前页,记录数,总记录数......)
* 2.为分页对象进行初始化操作
* 3.从分页操作中获取有效数据之后返回
* @param page
* @param rows
* @return
*/
@Override
public EasyUITable findItemByPage(Integer page, Integer rows) {
//1.定义分页对象 之后进行查询,
IPage<Item> iPage = new Page<>(page,rows);
//2.获取分页对象的其他数据
QueryWrapper<Item> queryWrapper = new QueryWrapper<>();
queryWrapper.orderByDesc("updated");
iPage = itemMapper.selectPage(iPage,queryWrapper);
long total = iPage.getTotal(); //获取总记录数
List<Item> itemList = iPage.getRecords(); //获取当前页的数据
return new EasyUITable(total,itemList);
}`
```
### 2.4.2 编辑分页配置类
```
`@Configuration //标识配置文件
public class MybatisPlusConfig {
@Bean //将数据交给spring容器管理
public PaginationInterceptor paginationInterceptor() {
PaginationInterceptor paginationInterceptor = new PaginationInterceptor();
// 设置请求的页面大于最大页后操作, true调回到首页,false 继续请求 默认false
paginationInterceptor.setOverflow(true);
// 设置最大单页限制数量,默认 500 条,-1 不受限制
// paginationInterceptor.setLimit(500);
// 开启 count 的 join 优化,只针对部分 left join
paginationInterceptor.setCountSqlParser(new JsqlParserCountOptimize(true));
return paginationInterceptor;
}
}`
```
2.5 数据格式化
---------
### 2.5.1 格式化价格
1).检查html代码
```
`<th data-options="field:'price',width:70,align:'right',formatter:KindEditorUtil.formatPrice">价格</th>`
```
2).检查函数
```
`/**
* val代表是当前节点的值
row代表当前的行级元素信息 对象
*/
// 格式化价格
formatPrice : function(val,row){
return (val/100).toFixed(2);
},`
```
### 2.5.2 格式化时间
1).编辑HTML代码
```
`<th data-options="field:'created',width:130,align:'center',formatter:KindEditorUtil.formatDateTime">创建日期</th>`
```
2).编辑页面JS
```
`// 格式化时间
formatDateTime : function(val,row){
//将字符串转化为js对象 时间对象
var now = new Date(val);
//将当前时间按照指定的格式进行转化
return now.format("yyyy-MM-dd hh:mm:ss");
},`
```
### 2.5.3 格式化商品状态
1).编辑HTML页面
```
`<th data-options="field:'status',width:60,align:'center',formatter:KindEditorUtil.formatItemStatus">状态</th>`
```
2).编辑页面JS
```
`// 格式化商品的状态
formatItemStatus : function formatStatus(val,row){
if (val == 1){
return '<span>上架</span>';
} else if(val == 2){
return '<span>下架</span>';
} else {
return '<span>未知</span>';
}
},`
```
### 2.5.4 JS引入过程
1).在父级页面中index.jsp中添加如下操作
```
`<jsp:include page="/commons/common-js.jsp"></jsp:include>`
```
2).检查common-js
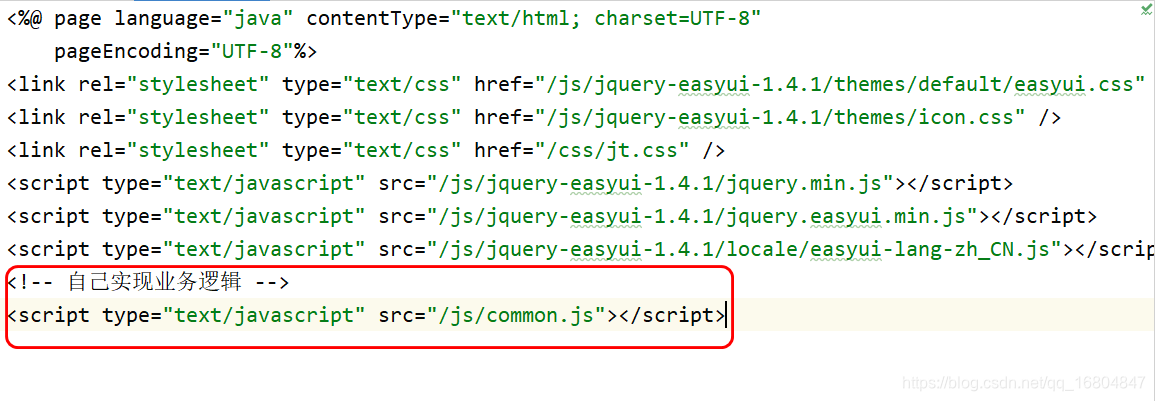
3.实现分类操作
========
3.1 实现商品分类回显
------------
### 3.1.1 编辑ItemCat对象
说明: 在jt-common中添加pojo对象
```
`package com.jt.pojo;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import lombok.Data;
import lombok.experimental.Accessors;
@TableName("tb_item_cat")
@Data
@Accessors(chain = true)
public class ItemCat extends BasePojo{
@TableId(type = IdType.AUTO)
private Long id; //商品分类id 主键自增
private Long parentId; //定义父级分类Id
private String name; //商品分类名称
private Integer status; //指定状态 1正常 2删除
private Integer sortOrder; //排序号
private Boolean isParent; //是否为父级
}`
```
### 3.1.1 页面标识
1).编辑html
```
`<th data-options="field:'cid',width:100,align:'center',formatter:KindEditorUtil.findItemCatName">叶子类目</th>`
```
2).编辑JS
```
`/**
* 完成$.ajax业务调用
属性说明:
1.type : 定义请求的类型 GET/POST/PUT/DELETE
2.URL: 指定请求的路径
3.dataType: 指定返回值类型 一般可以省略
4.data : ajax向后端服务器传递的数据
1.{key:value,key2:value2}
2.key=value&key2=value2
5.success: 设定回调函数一般都会携带参数
6.async 异步操作 默认值为true 改为false表示同步操作.
7.error 请求异常之后 执行的函数.
8.cache ajax是否使用缓存 默认值为true
1.动态获取用户传递的itemCatId的值.
2.利用ajax实现数据的动态的获取
ajax: $.get/$.post/$.getJSON/$.ajax
**/
findItemCatName : function(val,row){
//console.log("商品分类ID号:"+val);
let name = null;
// //异步出现了问题 由于异步操作程序没有返回数据之前,提前结束所以程序为null
$.ajax({
type : "GET",
url : "/itemCat/findItemCatById",
data : {id:val},
success : function(result){ //ItemCat
//console.log(result.name);
name = result.name;
},
async : false //设置为同步操作 true为异步....
})
return name;
},`
```
### 3.1.2 编辑ItemCatController
```
`package com.jt.controller;
import com.jt.pojo.ItemCat;
import com.jt.service.ItemCatService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/itemCat")
public class ItemCatController {
@Autowired
private ItemCatService itemCatService;
/**
* 实现商品分类查询
* url地址: http://localhost:8091/itemCat/findItemCatById?id=163
* 参数: id=163
* 返回值结果: itemCat对象
*/
@RequestMapping("/findItemCatById")
public ItemCat findItemCatById(Long id){
return itemCatService.findItemCatById(id);
}
}`
```
### 3.1.3 编辑ItemCatService
```
`package com.jt.service;
import com.jt.mapper.ItemCatMapper;
import com.jt.pojo.ItemCat;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class ItemCatServiceImpl implements ItemCatService{
@Autowired
private ItemCatMapper itemCatMapper;
@Override
public ItemCat findItemCatById(Long id) {
return itemCatMapper.selectById(id);
}
}`
```
### 3.1.4 页面效果展现
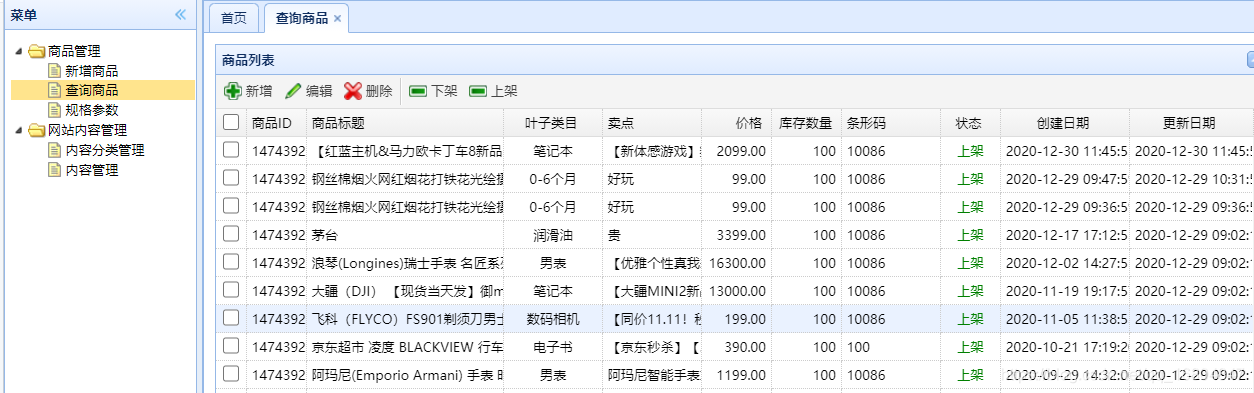
以上是 【Java】京淘项目Day04 的全部内容, 来源链接: utcz.com/a/110590.html
得票时间